Preface
How to understand scope? First we need to understand what a domain is.
Domain is a broad concept that has different meanings in different fields. In mathematics, a field is an algebraic structure that contains addition and multiplication and satisfies some specific properties, such as the closure of addition and multiplication, the associative law, the commutative law, the existence of unit elements and inverse elements, etc.
In computer science, a domain usually refers to a specific field or scope. For example, on the Internet, a domain name (Domain Name) is the name used to identify a network resource or a group of network resources. In addition, in the database, a domain refers to a set of attributes or fields, which defines the value range and constraints of the field.
In other fields, a domain may refer to a specific area of knowledge or activity, such as the field of electromagnetic fields in physics, or the market domain in business, etc. In general, a domain can refer to a range, area, or area within which there are specific rules, properties, or characteristics.
The scope of JavaScript refers to the area in the code where variables are defined, which determines the visibility and accessibility of variables in the code. Today we will introduce the global scope, function scope and block-level scope in JS
text
First the browser engine needs to perform lexical analysis on our code
When we enter this line of code into the compiler var a = 2
, the browser will analyze what lexical units are in this line of code. In this line of code, the tokens are var,a,=,2
After completing the lexical analysis, our code will be parsed
Parsing is to convert the lexical unit into a program syntax structure tree nested level by level.
The last step is to generate the code :var a = 2
- What is global scopeLet’s look at two pieces of code first
function foo(a){
console.log (a+b);
}
var b = 2
foo(1)
We can see that the result of this code is 3

function foo(a){
console.log (a+b);
}
function bar(){
var b = 2
}
bar()
foo(1)
We re-declare the bar function and declare b=2 in it. When running the code, an error is displayed. Why is this?
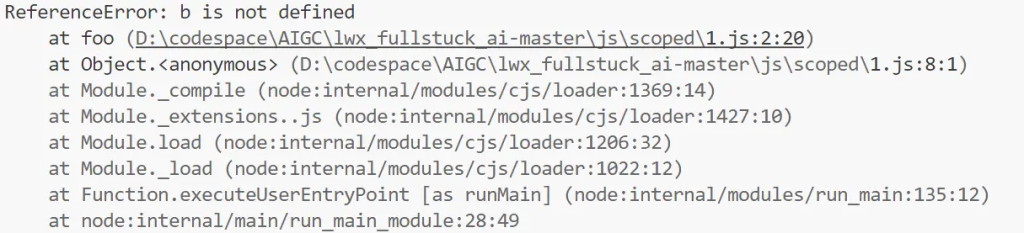
What is the difference between these two codes?
In the first code, we declare b globally, and in the second code, we declare it in the second function body.
We need to first understand what a valid identifier is: a variable declared within a function or a parameter within the function itself is the valid identifier of the function body, that is, something meaningful in a space.
Within the entire scope, in JavaScript, the global scope refers to the scope that can be accessed from anywhere in the code. In a browser environment, the global scope usually refers to window
an object, and in a Node.js environment it refers to global
an object.
- function scope
- The inner scope can access the outer scope, but not vice versa.
function foo() {
var a = 1
}
foo()
console.log(a);
a is within the scope of foo(), and the global scope is outside the scope of foo, so a cannot be found globally, so it cannot be printed.
At this time, we bring up a and declare it globally. Let’s print it and take a look.
var a = 1
function foo() {
console.log(a);
}
foo()

Because it is in the global scope, and the inner scope can access the outer scope, it can print out the value of a.
- block scope { let }
let VS var
1. There is a declaration promotion of var, but let does not exist.
2.let will form a block-level scope with {}
3.var can declare variables repeatedly, but let cannot
Summarize
In JavaScript, scoping is one of the core concepts in understanding the language. By understanding the principles of scope, we can better organize and manage our code to avoid variable conflicts and unexpected behavior. Whether it is global scope or function scope, they provide us with flexibility and control, allowing us to write clearer and more robust code.
When writing JavaScript code, keeping the importance of scope in mind, using global and local variables rationally, and avoiding variable pollution and unexpected side effects will help improve the maintainability and readability of the code. Scope is not only a language feature, but also a programming philosophy that guides us in writing high-quality, reliable JavaScript code.