Dart 3.2, released this time following Flutter 3.16 , includes: non-null improvements to private final fields, new interop improvements, extended support for DevTools, and updates to the Web roadmap, including support for Wasm’s Web components.
The most important thing is Wasm’s Web component support.
non-null type promotion of private final
Since the release of sound null safety in Dart 2.12, type promotion has been one of the core parts of null safety, but it is limited to local variables. Fields and top-level variables cannot be processed. For example, an error will be reported in this case:
class Container {
final int? _fillLevel;
Container(this._fillLevel);
check() {
if (_fillLevel != null) {
int i = _fillLevel; // Prior to Dart 3.2, causes an error.
}
}
}
This limitation is due to several complex situations where flow analysis cannot determine when a field will change, for example, in the case of field promotion, if a subclass overrides the field using a getter (which sometimes returns null), this may cause problems.
Starting with Dart 3.2, Dart has improved flow analysis and can now classify private Final fields .
Now the above code snippet in 3.2 can successfully pass the detection: for private & final fields, its value will never change after the initial allocation, so it is considered safe to check it only once.
New code analysis options in package: lints 3.0
3.2 also includes some improvements to the standard code analysis rules in package:lints , which contains the default and recommended set of static analysis rules that are provided with new projects created with dart create
or (via package:flutter_lints ).flutter create
The main version of the lint set (3.0) has been released, which adds 6 lints to the core set and 2 lints to the recommended set. It has related functions for validating pubspec URLs, verifying whether collection methods are called with correct parameters, etc. lints.
For a full list of changes, check out github.com/dart-lang/l… , version 3.0 will become the default version for new projects that will be released soon.
Dart interop updates
Efforts are currently underway to extend Dart interop to fully support direct calls with Java and Kotlin and Objective C and Swift , with a number of improvements starting in Dart 3.2:
- Introduced C FFI’s constructor
NativeCallable.isolateLocal
, which can create a C function pointer from any Dart function,Pointer.fromFunction
an extension of the functionality provided by C FFI, which can only create function pointers from top-level functions. - Updated Objective-C Utilization
NativeCallable.listener
Binding Generators that can now automatically handle APIs that contain asynchronous callbacks, such as Core Motion, code that previously required manual binding. - Improved package:jnigen to implement direct call support for Java and Kotlin, we are now able to migrate package:cronet_http (the wrapper for the Android Cronet HTTP client) from hand-written binding code to an automatically generated wrapper.
- Significant progress has been made on the Native Assets feature, which is designed to solve many problems related to the distribution of Dart packages that rely on Native code, by providing unified hooks to the various build needs involved in building Flutter and standalone Dart apps. , details can be found at: dart.dev/guides/libr…Native Assets is currently an experimental feature that allows Dart packages to more seamlessly depend on and use Native code, building and bundling Native code through
flutter run
/flutter build
anddart run
/ .dart build
Note: Can be enabled viaflutter config --enable-native-assets
andflutter create --template=package_ffi [package name]
.
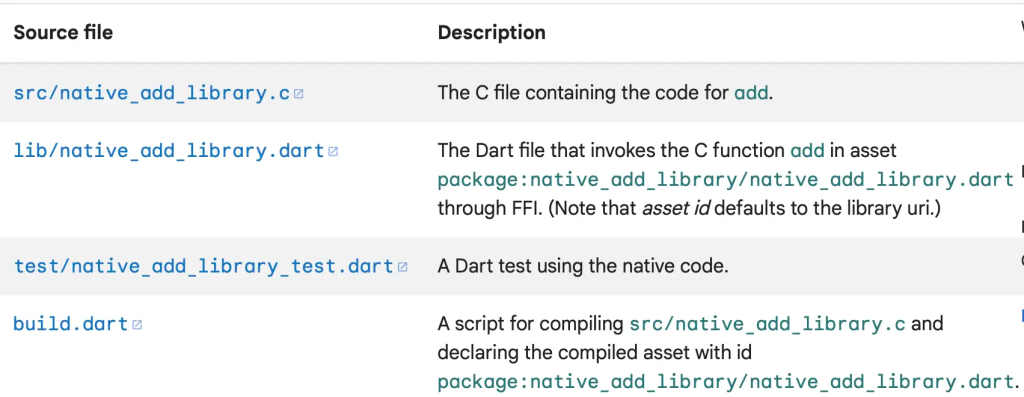
- Demo
native_add_library
shows related usage. When the Flutter project depends onpackage:native_add_library
, the script will automaticallybuild.dart
be called on the command:import 'package:native_add_library/native_add_library.dart'; void main() { print('Invoking a native function to calculate 1 + 2.'); final result = add(1, 2); print('Invocation success: 1 + 2 = $result.'); }
DevTools extension for Dart packages
A new extension framework has been released in Dart 3.2 and Flutter 3.16 , which allows package authors to build custom tools for their packages and display them directly in DevTools.
It allows the pub.dev package containing the framework to provide custom tools for specific use cases. For example, the author of Serverpod has been working hard to build developer tools for its package and is excited to provide the DevTools extension in the upcoming 1.2 release .
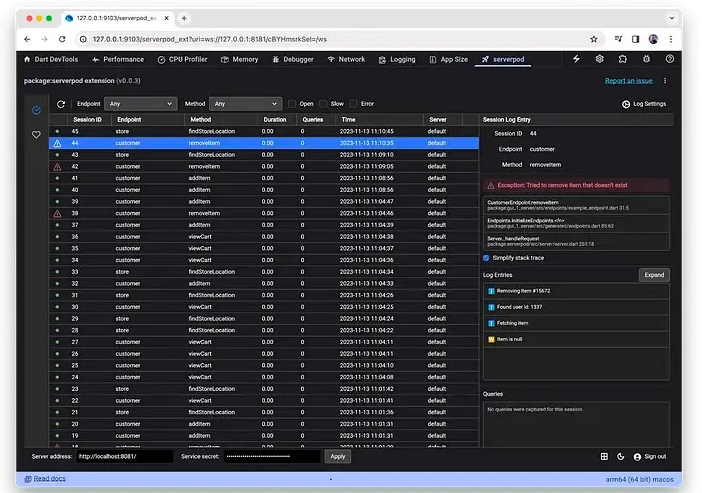
Dart Web and Wasm updates
Starting from Chrome 119, Chrome will enable Wasm garbage collection support (called Wasm-GC) by default . Wasm-GC support also appears in Firefox 120 (their next stable version) and is supported, then Dart, Flutter and Wasm-GC What is the current situation?
The Dart-to-Wasm compiler is almost fully functional and the team is very pleased with the performance and compatibility, now focusing on edge cases to ensure it works equally well in a wide range of scenarios.
For Flutter Web, this is similar to the completion of a brand new “Skwasm” rendering engine. In order to maximize performance, Skwasm connects the compiled application code directly to the custom CanvasKit Wasm module through wasm-to-wasm binding . This is also the first iteration of Flutter Web multi-threaded rendering support, further improving the frame rate. time.
Before Wasm’s Flutter web is ready to leave its current experimental status, there are still a few things to do:
- Dual Compilation : Generates Wasm and JavaScript output and enables feature detection at runtime to support browsers with and without Wasm-GC support.
- JavaScript interop : A new JS interop mechanism based on extended types that, when targeting JavaScript and Wasm, enables concise, type-safe calls between Dart code, browser APIs, and JS libraries.
- Browser API with Wasm support : A new
package:web
, modern JS-based interop mechanism replaces dart:html (and related libraries), which will provide easier access to the browser API and support JS and Wasm targets.
We have already started migrating some internal projects to package:web
the new JS interop mechanism, and expect more updates in the next stable version.
You can learn more at flutter.dev/wasm .
at last
There are two most important points in this update. The first is that Dart interop is becoming more and more mature. I believe that in the future, all interop binding and compilation can be completed directly through flutter run. The second is that with the progress of Dart Wasm support for the Web route, It’s becoming more and more worth looking forward to.